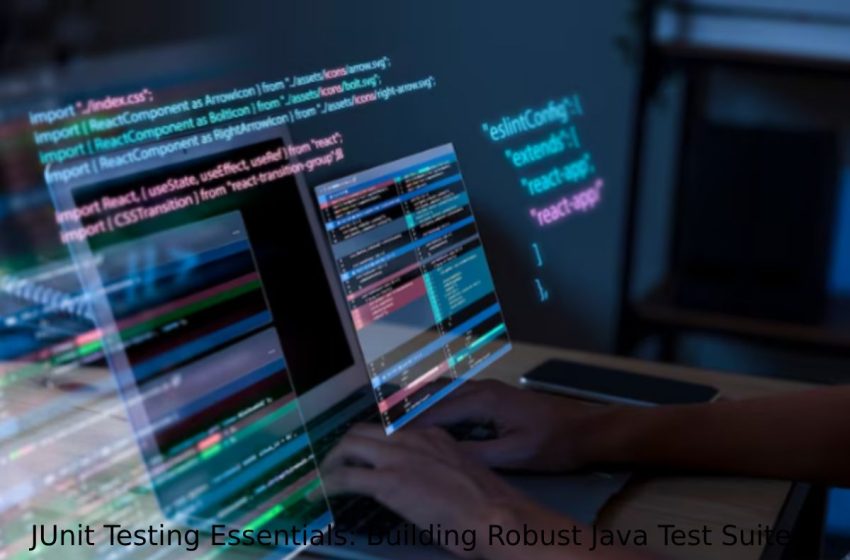
JUnit Testing Essentials: Building Robust Java Test Suites
JUnit is currently considered one of the most utilized testing solutions in the Java environment, offering numerous features that facilitate developers’ proper development of tests. Rescue techniques using JUnit for constructing robust test suites can dramatically improve the quality of your Java applications by guaranteeing that alterations to applications do not come to include new faults and that applications operate as suitable.
Understanding the JUnit framework is essential for effective testing, particularly when focusing on test suites, the characteristics of JUnit testing, and practical considerations for organizing tests efficiently.
Table of Contents
Introduction to JUnit
JUnit is specially designed for unit tests. Java is a language that allows programmers to write test cases and run them repeatedly. It is a collaborative project of the JUnit project and has a special relevance to TDD and BDD. JUnit has been developed with the primary objective of making available a toolbox that could help ensure that the codes used in Java-based applications are correct.
JUnit allows the definition of test cases as Java methods so that when the program is run, the developer simply invokes the method in order to check its behavior. Besides, through automating it, not only does it take less time, but the test itself can also be of a higher quality due to decreased human factors influence.
Setting Up JUnit
Before introducing JUnit, there is something you need to do with your environment of development. Here’s how to do it:
- Include JUnit in Your Project: The only tool that needs to be used in order to include JUnit in your project is a build tool such as Maven or Gradle, which is very easy to use. For instance, Maven needs to include the following in your `pom.xml`:
“`xml
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
“`
For Gradle, add the following to your `build.gradle`:
“`groovy
testImplementation ‘org.junit.jupiter:junit-jupiter:5.8.1’
“`
- Configure Your IDE: For now, any contemporary IDE, including IntelliJ IDEA or Eclipse, supports JUnit as a testing framework. Ensure that your IDE is configured to identify JUnit tests and execute them effortlessly.
- Create Your First Test Class: To begin with, writing tests, create a new java class in your test source folder, which would mostly be src/test/java, and at the top of the java file, it should be tagged as @Test.
Core Annotations in JUnit
JUnit provides several annotations that help define test behavior. Understanding these annotations is crucial for building compelling test suites:
- @Test: Marks a method as a test method.
- @BeforeEach: Executed before each test method. Use this to set up standard test fixtures.
- @AfterEach: Executed after each test method. Use this for cleanup.
- @BeforeAll: Executed once before all tests in the class. This method must be static.
- @AfterAll: Executed once after all tests in the class. This method must be static.
- @Disabled: Marks a test as ignored (not executed).
- @DisplayName: Allows you to provide a custom name for your test methods.
Writing Test Cases
Writing test cases in JUnit is straightforward. Below is a description of one example of the test case in basic calculator class:
“`java
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
private Calculator calculator;
@BeforeEach
public void setUp() {
calculator = new Calculator();
}
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3));
}
@Test
public void testSubtract() {
assertEquals(1, calculator.subtract(3, 2));
}
}
“`
In this example:
- Setup: The setUp() method initializes a new instance of the `Calculator` class before each test.
- Assertions: The assertEquals() method checks if the expected result matches the actual result returned by the methods in the Calculator()
Best Practices for Organizing Test Suites
To build robust test suites, consider the following best practices:
- Structure Your Tests: Organize your test classes meaningfully. Group related tests together and keep your test classes focused on specific functionality.
- Use Descriptive Names: Be very thoughtful in selecting names for your test methods, giving names that depict the tested behavior. This makes it easier to understand pre-test goals and objectives since the tests seem much more exquisite in design.
- Keep Tests Independent: Make sure that no two tests are consecutive. To arrive at an apt conclusion, each test should be capable of running independently to avoid an instance where one failure leads to the others.
- Use Assertions Wisely: Assertions should be used to confirm expected results as a form of documentation. Be specific in your assertions to ensure clarity in what is being tested.
- Document Your Tests: Use comments or Javadoc to explain complex test cases. This will help other developers (and your future self) understand the purpose of the tests.
- Prioritize Reusability: Write reusable utility functions or helper methods for common test actions, such as setting up test data or handling environment configurations. This minimizes redundancy, reduces maintenance effort, and enhances consistency.
- Implement Setup and Teardown Methods: Use setup and teardown methods to prepare the testing environment and clean up afterward. By centralizing initialization and cleanup routines, you ensure that each test starts with a clean state and avoids unintended side effects.
- Limit External Dependencies: Avoid reliance on external services or databases when possible, as they can introduce instability. Consider using mocks or stubs to simulate responses, ensuring that tests run consistently and independently of external changes.
Advanced Testing Techniques
As your testing needs grow, you may want to explore advanced techniques:
- Parameterized Tests: Use parameterized tests to run the same test with different input values. This helps reduce code duplication.
“`java
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.MethodSource;
public class ParameterizedCalculatorTest {
@ParameterizedTest
@MethodSource(“provideNumbersForAddition”)
void testAdd(int a, int b, int expected) {
assertEquals(expected, calculator.add(a, b));
}
static Stream<Arguments> provideNumbersForAddition() {
return Stream.of(
Arguments.of(1, 2, 3),
Arguments.of(2, 3, 5),
Arguments.of(0, 0, 0)
);
}
}
“`
- Mocking: Using dependencies in your tests to create mock objects must be done with the help of mocking frameworks such as Mockito. This enables you to domesticate your code being tested, hence fingerprinting its behavior.
Integrating JUnit with Build Tools
Integrating JUnit with your build tool enhances your testing process. Here’s how to do it with Maven and Gradle:
- Maven: By default, Maven knows that all test classes are stored in the `src/test/java` directory and executes these tests during the test stage. In addition to running integration tests against a database, you can configure the `maven-surefire-plugin` to manage the execution of tests.
- Gradle: Gradle also detects and runs tests automatically. You can configure test tasks in your `build.gradle` file for customized behavior, such as specifying test sources or test report outputs.
Mocking and Stubbing with JUnit
When your tests depend on external components (like databases or web services), mocking becomes essential. Here’s how to use Mockito with JUnit:
- Add Mockito Dependency: Include Mockito in your build file. For Maven, add:
“`xml
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>4.0.0</version>
<scope>test</scope>
</dependency>
“`
- Create Mocks: Use Mockito to create mock objects in your tests:
“`java
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class ServiceTest {
@Test
void testServiceMethod() {
Repository mockRepo = mock(Repository.class);
when(mockRepo.getData()).thenReturn(new Data());
Service service = new Service(mockRepo);
// Call service methods and assert results
}
}
“`
Continuous Integration and JUnit
Automated testing using JUnit is common in most Continuous Integration (CI) processes. Here’s how to set it up:
- Choose a CI Tool: Choose a CI tool from Jenkins, Travis CI, or GitHub Actions.
- Configure Your CI Pipeline: Set up your CI configuration file to include JUnit test execution. This typically involves defining steps to build your project and run tests.
- Monitor Test Results: Ensure that your CI pipeline provides feedback on test results. Most CI tools will report the status of your tests, allowing you to catch failures early in the development process.
Best Practices for JUnit Testing
To ensure that your JUnit tests are effective, follow these best practices:
1. Keep Tests Independent
Each test should be a single test to prevent a failure cascade. This means that the success or failure of one test cannot influence the others, easing debugging and increasing reliability in the result. Independent tests also provide clearer insights into which parts of the code are problematic.
2. Use Descriptive Names
Use descriptive names for test methods to convey the purpose clearly. A well-named test method should immediately inform the developer of the behavior it is testing. It is particularly useful when reviewing test results or working in large teams, as it aids in quick understanding and better collaboration.
3. Test One Thing at a Time
Each test should focus on a single behavior to simplify debugging. By isolating the behavior you’re testing, you can quickly identify the root cause of failures and ensure that specific functionality is being thoroughly validated without interference from other code.
4. Use SetUp and TearDown Wisely
Utilize @BeforeEach and @AfterEach for common setup and cleanup tasks. It ensures that any required resources, configurations, or states are properly initialized before each test and cleaned up afterward. It helps avoid repetitive code and ensures a consistent environment for all tests.
5. Keep Tests Fast
Aim for tests that run quickly to facilitate frequent execution. Fast tests encourage regular testing during development and ensure that CI/CD pipelines run efficiently. Avoid external dependencies or heavy computations that could slow down the test execution.
6. Document Test Cases
Use comments to clarify complex tests, making them easier to understand. Well-documented tests also help other developers grasp the purpose of the test and the logic behind it. It is especially important for complex logic or edge cases, reducing future maintenance efforts.
7. Cross-Browser Testing with Cloud-Based Tools
Cross-browser testing tools such as LambdaTest can be useful for better results with JUnit testing. If you are able to perform your test suites on multiple browsers and devices, you will be able to identify potential issues that different users might encounter, ensuring a consistent experience for all. This practice is especially important in today’s diverse web landscape, where users access applications from various browsers and platforms.
With platforms like LambdaTest, you can conduct parallel testing across more than 3,000 browsers and operating systems. Whether you’re running Selenium Java-based tests or using another framework, this ensures that your application performs exactly as expected, regardless of the user’s configuration.
In Conclusion
JUnit offers a perfect solution for creating elaborate and extensive test languages in Java. When thinking about test organization, some of the lessons that can be followed include Avoiding creating tests in ways that depend on another test, using meaningful names for the tests, and advancing features like parameterized tests and mock tests. Furthermore, using tools such as LambdaTest for cross-browser testing helps improve your campaign strategy by pointing out the places where your application may unexpectedly act incompatibly and addressing these issues as early as possible.
Even with all the advances in DevOps and CI/CD, JUnit has high relevancy now, as it is lightweight, easy to integrate with most modern CI and CD pipelines, and powerful in the hands of a dedicated developer who wants to maintain high code quality and deliver bug-free software to the client or customer. Approaching the creation of test suites in a proper and structured way and continuously using the described techniques and best practices, you will be set up well for creating long-term, reliable, and scalable Java test suites.